
Building RESTful Web Services with Java EE 8
Create modern RESTful web services with the Java EE 8 API
Mario-Leander Reimer
- 116 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Building RESTful Web Services with Java EE 8
Create modern RESTful web services with the Java EE 8 API
Mario-Leander Reimer
About This Book
Learn the fundamentals of Java EE 8 APIs to build effective web services
Key Features
- Design modern and stylish web services with Java EE APIs
- Secure your web services with JSON Web Tokens
- Explore the advanced concepts of RESTful web services and the JAX-RS API
Book Description
Java Enterprise Edition is one of the leading application programming platforms for enterprise Java development. With Java EE 8 finally released and the first application servers now available, it is time to take a closer look at how to develop modern and lightweight web services with the latest API additions and improvements.
Building RESTful Web Services with Java EE 8 is a comprehensive guide that will show you how to develop state-of-the-art RESTful web services with the latest Java EE 8 APIs. You will begin with an overview of Java EE 8 and the latest API additions and improvements. You will then delve into the details of implementing synchronous RESTful web services and clients with JAX-RS. Next up, you will learn about the specifics of data binding and content marshalling using the JSON-B 1.0 and JSON-P 1.1 APIs.
This book also guides you in leveraging the power of asynchronous APIs on the server and client side, and you will learn to use server-sent events (SSEs) for push communication. The final section covers advanced web service topics such as validation, JWT security, and diagnosability.
By the end of this book, you will have implemented several working web services and have a thorough understanding of the Java EE 8 APIs required for lightweight web service development.
What you will learn
- Dive into the latest Java EE 8 APIs relevant for developing web services
- Use the new JSON-B APIs for easy data binding
- Understand how JSON-P API can be used for flexible processing
- Implement synchronous and asynchronous JAX-RS clients
- Use server-sent events to implement server-side code
- Secure Java EE 8 web services with JSON Web Tokens
Who this book is for
If you're a Java developer who wants to learn how to implement web services using the latest Java EE 8 APIs, this book is for you. Though no prior knowledge of Java EE 8 is required, experience with a previous Java EE version will be beneficial.
Frequently asked questions
Information
Building Synchronous Web Services and Clients
- Implementing basic REST APIs with JAX-RS
- Using sub-resources
- Error handling in JAX-RS
- Implementing web service clients with Java EE 8
- Testing Java EE 8 web services
Implementing basic REST APIs with JAX-RS

@Path("books")
@RequestScoped
public class BookResource {
@Inject
private Bookshelf bookshelf;
@GET
@Produces(MediaType.APPLICATION_JSON)
public Response books() {
return Response.ok(bookshelf.findAll()).build();
}
@GET
@Path("/{isbn}")
public Response get(@PathParam("isbn") String isbn) {
Book book = bookshelf.findByISBN(isbn);
return Response.ok(book).build();
}
@POST
@Consumes(MediaType.APPLICATION_JSON)
public Response create(Book book) {
if (bookshelf.exists(book.getIsbn())) {
return Response.status(Response.Status.CONFLICT).build();
}
bookshelf.create(book);
URI location = UriBuilder.fromResource(BookResource.class)
.path("/{isbn}")
.resolveTemplate("isbn", book.getIsbn())
.build();
return Response.created(location).build();
}
@PUT
@Path("/{isbn}")
public Response update(@PathParam("isbn") String isbn, Book book) {
bookshelf.update(isbn, book);
return Response.ok().build();
}
@DELETE
@Path("/{isbn}")
public Response delete(@PathParam("isbn") String isbn) {
bookshelf.delete(isbn);
return Response.ok().build();
}
FROM payara/micro:5-SNAPSHOT
COPY target/library-service.war /opt/payara/deployments
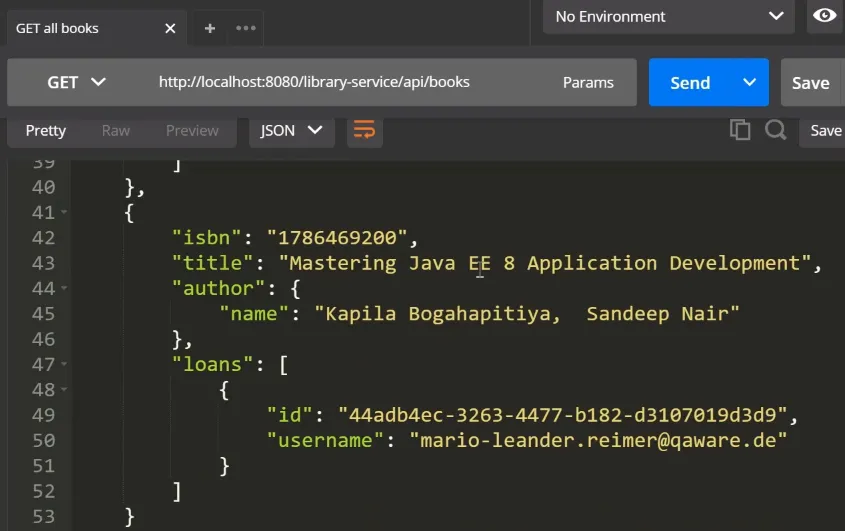

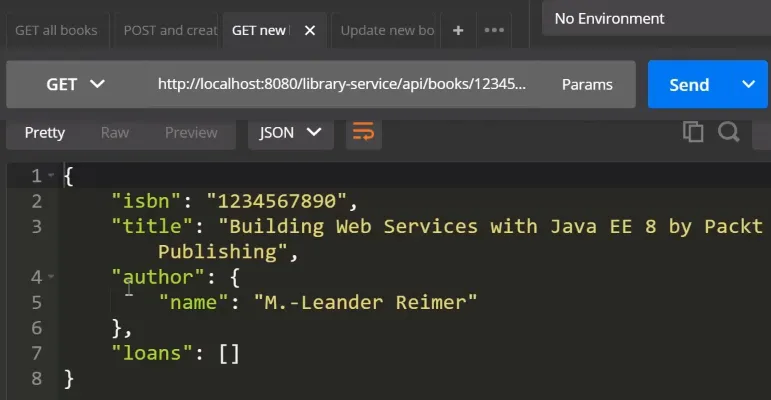
Using sub-resources

