
Python Artificial Intelligence Projects for Beginners
Get up and running with Artificial Intelligence using 8 smart and exciting AI applications
Dr. Joshua Eckroth
- 162 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Python Artificial Intelligence Projects for Beginners
Get up and running with Artificial Intelligence using 8 smart and exciting AI applications
Dr. Joshua Eckroth
About This Book
Build smart applications by implementing real-world artificial intelligence projects
Key Features
- Explore a variety of AI projects with Python
- Get well-versed with different types of neural networks and popular deep learning algorithms
- Leverage popular Python deep learning libraries for your AI projects
Book Description
Artificial Intelligence (AI) is the newest technology that's being employed among varied businesses, industries, and sectors. Python Artificial Intelligence Projects for Beginners demonstrates AI projects in Python, covering modern techniques that make up the world of Artificial Intelligence.
This book begins with helping you to build your first prediction model using the popular Python library, scikit-learn. You will understand how to build a classifier using an effective machine learning technique, random forest, and decision trees. With exciting projects on predicting bird species, analyzing student performance data, song genre identification, and spam detection, you will learn the fundamentals and various algorithms and techniques that foster the development of these smart applications. In the concluding chapters, you will also understand deep learning and neural network mechanisms through these projects with the help of the Keras library.
By the end of this book, you will be confident in building your own AI projects with Python and be ready to take on more advanced projects as you progress
What you will learn
- Build a prediction model using decision trees and random forest
- Use neural networks, decision trees, and random forests for classification
- Detect YouTube comment spam with a bag-of-words and random forests
- Identify handwritten mathematical symbols with convolutional neural networks
- Revise the bird species identifier to use images
- Learn to detect positive and negative sentiment in user reviews
Who this book is for
Python Artificial Intelligence Projects for Beginners is for Python developers who want to take their first step into the world of Artificial Intelligence using easy-to-follow projects. Basic working knowledge of Python programming is expected so that you're able to play around with code
Frequently asked questions
Information
Applications for Comment Classification
Text classification
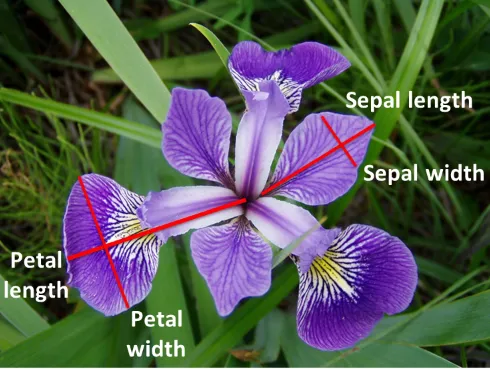

Machine learning techniques
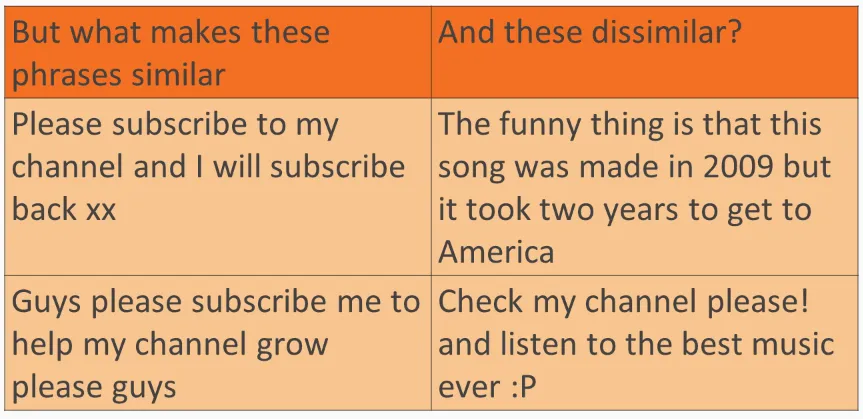
Bag of words


- Lowercase every word
- Drop punctuation
- Drop very common words (stop words)
- Remove plurals (for example, bunnies => bunny)
- Perform lemmatization (for example, reader => read, reading = read)
- Use n-grams, such as bigrams (two-word pairs) or trigrams
- Keep only frequent words (for example, must appear in >10 examples)
- Keep only the most frequent M words (for example, keep only 1,000)
- Record binary counts (1 = present, 0 = absent) rather than true counts