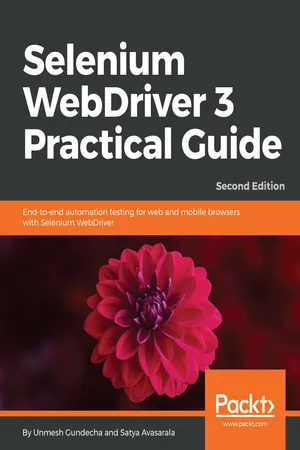
Selenium WebDriver 3 Practical Guide
End-to-end automation testing for web and mobile browsers with Selenium WebDriver, 2nd Edition
Unmesh Gundecha, Satya Avasarala
- 280 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Selenium WebDriver 3 Practical Guide
End-to-end automation testing for web and mobile browsers with Selenium WebDriver, 2nd Edition
Unmesh Gundecha, Satya Avasarala
About This Book
Real-world examples of cross-browser, mobile, and data-driven testing with all the latest features of Selenium WebDriver 3
Key Features
- Unlock the full potential of Selenium to test your web applications
- Use Selenium Grid for faster, parallel running, and cross-browser testing
- Test iOS and Android Apps with Appium
Book Description
Selenium WebDriver is an open source automation tool implemented through a browser-specific driver, which sends commands to a browser and retrieves results. The latest version of Selenium 3 brings with it a lot of new features that change the way you use and setup Selenium WebDriver. This book covers all those features along with the source code, including a demo website that allows you to work with an HMTL5 application and other examples throughout the book.
Selenium WebDriver 3 Practical Guide will walk you through the various APIs of Selenium WebDriver, which are used in automation tests, followed by a discussion of the various WebDriver implementations available. You will learn to strategize and handle rich web UI using advanced WebDriver API along with real-time challenges faced in WebDriver and solutions to handle them. You will discover different types and domains of testing such as cross-browser testing, load testing, and mobile testing with Selenium. Finally, you will also be introduced to data-driven testing using TestNG to create your own automation framework.
By the end of this book, you will be able to select any web application and automate it the way you want.
What you will learn
- Understand what Selenium 3 is and how is has been improved than its predecessor
- Use different mobile and desktop browser platforms with Selenium 3
- Perform advanced actions, such as drag-and-drop and action builders on web page
- Learn to use Java 8 API and Selenium 3 together
- Explore remote WebDriver and discover how to use it
- Perform cross browser and distributed testing with Selenium Grid
- Use Actions API for performing various keyboard and mouse actions
Who this book is for
Selenium WebDriver 3 Practical Guide is for software quality assurance/testing professionals, software project managers, or software developers interested in using Selenium for testing their applications. Prior programming experience in Java is necessary.
Frequently asked questions
Information
The PageObject Pattern
- What is the PageObject pattern design?
- Good practices for designing PageObjects
- Extensions to the PageObject pattern
- An end-to-end example
Creating test cases for our WordPress blog
Test case 1 – adding a new post to our WordPress blog
@Test
public void testAddNewPost() {
WebElement email = driver.findElement(By.id("user_login"));
WebElement pwd = driver.findElement(By.id("user_pass"));
WebElement submit = driver.findElement(By.id("wp-submit"));
email.sendKeys("admin");
pwd.sendKeys("$$SUU3$$N#");
submit.click();
// Go to AllPosts page
driver.get("http://demo-blog.seleniumacademy.com/wp/wp-admin/edit.php");
// Add New Post
WebElement addNewPost = driver.findElement(By.linkText("Add New"));
addNewPost.click();
// Add New Post's Content
WebElement title = driver.findElement(By.id("title"));
title.click();
title.sendKeys("My First Post");
driver.switchTo().frame("content_ifr");
WebElement postBody = driver.findElement(By.id("tinymce"));
postBody.sendKeys("This is description");
driver.switchTo().defaultContent();
// Publish the Post
WebElement publish = driver.findElement(By.id("publish"));
publish.click();
}
- Log into the WordPress Admin portal.
- Go to the All Posts page.
- Click on the Add New post button.
- Add a new post by providing the title and description.
- Publish the post.
Test case 2 – deleting a post from our WordPress blog
@Test
public void testDeleteAPost() {
WebElement email = driver.findElement(By.id("user_login"));
WebElement pwd = driver.findElement(By.id("user_pass"));
WebElement submit = driver.findElement(By.id("wp-submit"));
email.sendKeys("admin");
pwd.sendKeys("$$SUU3$$N#");
submit.click();
// Go to AllPosts page
driver.get("http://demo-blog.seleniumacademy.com/wp/wp-admin/edit.php");
// Click on the post to be deleted
WebElement post = driver.findElement(By.linkText("My First Post"));
post.click();
// Delete Post
WebElement publish = driver.findElement(By.linkText("Move to Trash"));
publish.click();
}
- Log into the WordPress Admin portal.
- Go to the All Posts page.
- Click...