
Mastering Qt 5
Create stunning cross-platform applications using C++ with Qt Widgets and QML with Qt Quick, 2nd Edition
Guillaume Lazar, Robin Penea
- 534 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Mastering Qt 5
Create stunning cross-platform applications using C++ with Qt Widgets and QML with Qt Quick, 2nd Edition
Guillaume Lazar, Robin Penea
About This Book
An In-depth guide updated with the latest version of Qt 5.11 including new features such as Quick Controls and Qt Gamepad
Key Features
- Unleash the power of Qt 5.11 with C++
- Build applications using Qt Widgets (C++) or Qt Quick (QML)
- Create cross-platform applications for mobile and desktop platforms with Qt 5
Book Description
Qt 5.11 is an app development framework that provides a great user experience and develops full capability applications with Qt Widgets, QML, and even Qt 3D. Whether you're building GUI prototypes or fully-fledged cross-platform GUI applications with a native look and feel, Mastering Qt 5 is your fastest, easiest, and most powerful solution. This book addresses various challenges and teaches you to successfully develop cross-platform applications using the Qt framework, with the help of well-organized projects.
Working through this book, you will gain a better understanding of the Qt framework, as well as the tools required to resolve serious issues, such as linking, debugging, and multithreading. You'll start off your journey by discovering the new Qt 5.11 features, soon followed by exploring different platforms and learning to tame them. In addition to this, you'll interact with a gamepad using Qt Gamepad. Each chapter is a logical step for you to complete in order to master Qt.
By the end of this book, you'll have created an application that has been tested and is ready to be shipped.
What you will learn
- Create stunning UIs with Qt Widgets and Qt Quick 2
- Develop powerful, cross-platform applications with the Qt framework
- Design GUIs with the Qt Designer and build a library in it for UI previews
- Handle user interaction with the Qt signal or slot mechanism in C++
- Prepare a cross-platform project to host a third-party library
- Use the Qt Animation framework to display stunning effects
- Deploy mobile apps with Qt and embedded platforms
- Interact with a gamepad using Qt Gamepad
Who this book is for
Mastering Qt 5 is for developers and programmers who want to build GUI-based applications. C++ knowledge is necessary, and knowing QT basics will help you get the most out of this book.
Frequently asked questions
Information
Even Qt Deserves a Slice of Raspberry Pi
- Discovering Qt3D
- Configuring Qt for your Raspberry Pi
- Crafting entities from the factory
- Building a snake engine in JavaScript
- Profiling your QML application
Discovering Qt3D
- 2D and 3D rendering for C++ and Qt Quick
- Meshes
- Materials
- GLSL shaders
- Shadow mapping
- Deferred rendering
- Instance rendering
- Uniform Buffer Object
- A mesh component, holding the vertices of your apple
- A material component, applying a texture on the mesh or coloring it
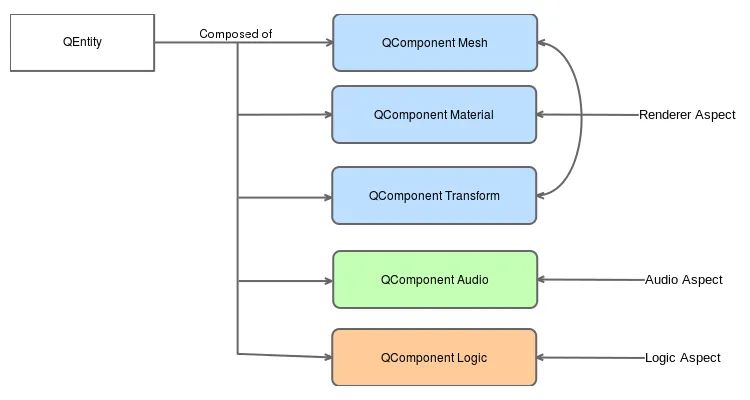
import Qt3D.Core 2.0 import Qt3D.Render 2.0 import Qt3D.Extras 2.0 Entity { property alias position: transform.translation PhongMaterial { id: material diffuse: "red" } SphereMesh { id: mesh } Transform { id: transform } components: [material, mesh, transform] }
- Entity: This is the root object of the file; it follows the same QML pattern we studied in Chapter 5, Dominating the Mobile UI.
- PhongMaterial: This defines how the surface will be rendered. Here, it uses the Phong shading technique to achieve smooth surfaces. It inherits QMaterial, which is the base class for all the material classes.
- SphereMesh: This defines what type of mesh will be used. It inherits QGeometryRenderer, which also gives the ability to load custom models (exported from 3D modeling software).
- Transform: This defines the transformation matrix of the component. It can customize the translation, scale, and position of the Entity element.
- position: This is a property to expose transform.translation for a given caller/parent. This might quickly become handy if we want to move the apple around.
- components: This is the array containing all the IDs of all the components for the Entity element.
import Qt3D.Core 2.0 import Qt3D.Render 2.0 import Qt3D.Extras 2.0 Entity { id: sceneRoot RenderSettings { id: renderSettings activeFrameGraph: ForwardRenderer { clearColor: Qt.rgba(0, 0, 0, 1) } } Apple { id: apple position: Qt.vector3d(3.0, 0.0, 2.0) } components: [frameGraph] }
import Qt3D.Core 2.0 import Qt3D.Render 2.0 import Qt3D.Extras 2.0
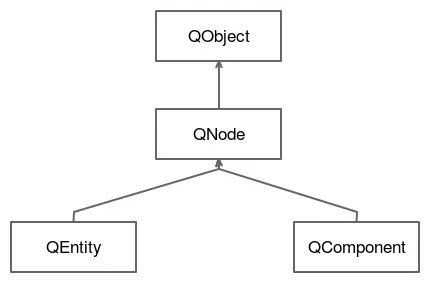
Rectangle { Scene3D { id: scene anchors.fill: parent focus: true World { } } }