
Selenium WebDriver Quick Start Guide
Write clear, readable, and reliable tests with Selenium WebDriver 3
Pinakin Chaubal
- 192 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Selenium WebDriver Quick Start Guide
Write clear, readable, and reliable tests with Selenium WebDriver 3
Pinakin Chaubal
About This Book
Get writing tests and learn to design your own testing framework with Selenium WebDriver API
Key Features
- Learn Selenium from the ground up
- Design your own testing framework
- Create reusable functionality in your framework
Book Description
Selenium WebDriver is a platform-independent API for automating the testing of both browser and mobile applications. It is also a core technology in many other browser automation tools, APIs, and frameworks. This book will guide you through the WebDriver APIs that are used in automation tests.
Chapter by chapter, we will construct the building blocks of a page object model framework as you learn about the required Java and Selenium methods and terminology.
The book starts with an introduction to the same-origin policy, cross-site scripting dangers, and the Document Object Model (DOM). Moving ahead, we'll learn about XPath, which allows us to select items on a page, and how to design a customized XPath. After that, we will be creating singleton patterns and drivers. Then you will learn about synchronization and handling pop-up windows. You will see how to create a factory for browsers and understand command design patterns applicable to this area.
At the end of the book, we tie all this together by creating a framework and implementing multi-browser testing with Selenium Grid.
What you will learn
- Understand what an XPath is and how to design a customized XPath
- Learn how to create a Maven project and build
- Create a Singleton driver
- Get to grips with Jenkins integration
- Create a factory for browsers
- Implement multi-browser testing with Selenium Grid
- Create a sample pop-up window and JavaScript alert
- Report using Extent Reports
Who this book is for
This book is for software testers or developers.
Frequently asked questions
Information
Hybrid Framework
- Introducing WebDriverManager
- DataProviders in TestNG
- TestNG listeners and assertions
- Incorporating logging and reporting in the framework
- Steps to add keywords to the framework
Technical requirements
https://github.com/PacktPublishing/Selenium-WebDriver-Quick-Start-Guide/tree/master/Chapter08
http://bit.ly/2O8KoXW
Introducing the WebDriverManager library
How to use the WebDriverManager library
- As a Java dependency
- As a command-line interface
- As a server in itself
WebDriverManager as a Java dependency
<dependency>
<groupId>io.github.bonigarcia</groupId>
<artifactId>webdrivermanager</artifactId>
<version>3.0.0</version>
<scope>test</scope>
</dependency>
private WebDriver driver = null;
System.setProperty("webdriver.chrome.driver", "C:\\chromedriver.exe");
driver = new ChromeDriver();
private WebDriver driver = null;
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
private WebDriver driver=null;
WebDriverManager.firefoxdriver().setup();
driver = new FirefoxDriver();
private WebDriver driver=null;
WebDriverManager.iedriver().setup();
driver = new InternetExplorerDriver();
public class BrowserFactory {
private WebDriver driver = null;
public void openBrowser(String browser) {
driver = null;
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
}
}
WebDriverManager as CLI
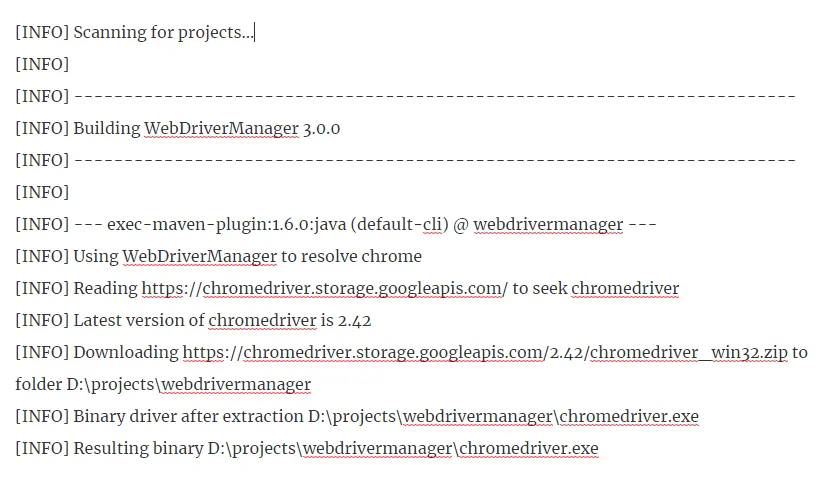
WebDriverManagerServer
- Directly from the code and Maven
- Using WebDriverManager as a fat JAR
- Directly from the code and Maven: The command to be used is: mvn exec:java -Dexec.args="server <port>". If the second argument is omitted, the default port (4041) will be used: C:\ Maven mvn exec:java -Dexec.args="server":
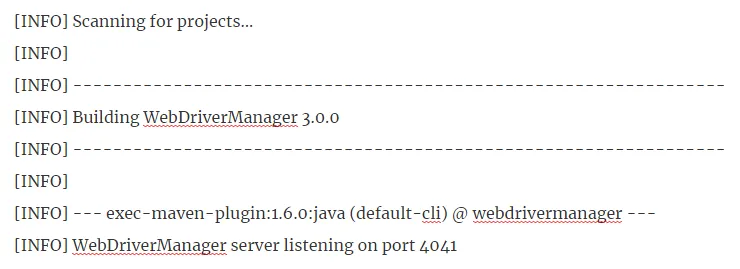
- Using WebDriverManager as a fat-jar: We can also use WebDriverManager as a fat JAR. For instance: java -jar webdrivermanager-3.0.0-fat.jar chrome.
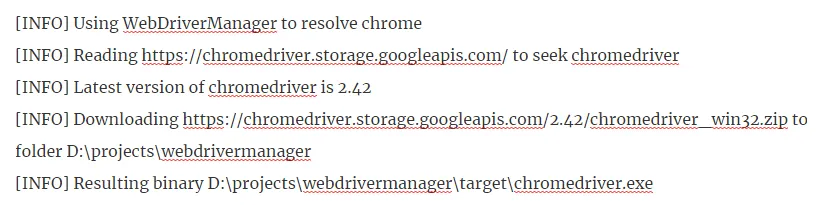
- http://localhost:4041/chromedriver: The latest version of chromedriver
- http://localhost:4041/firefoxdriver: The latest version of geckodriver
- http://localhost:4041/operadriver: The latest version of operadriver
- http://localhost:4041/phantomjs: The latest version of phantomjs driver
- http://localhost:4041/edgedriver: The latest version of MicrosoftWebDriver
- http://localhost:4041/iedriver: The latest version of IEDriverServer
Advantages of WebDriverManager
- It checks the browser version installed on your machine (for example, Chrome, Firefox, Internet Explorer, and so on).
- It checks the version of the driver (for example, chromedriver, geckodriver). If the version is not known, it uses the latest version of the driver.
- It downloads the WebDriver binary executable if it is not present in the WebDriverManager cache in the Maven repository (~/.m2/repository/webdriver by default).
- It exports the appropriate WebDriver Java environment variables required by Selenium (not done when WebDriverManager is used from the the command-line interface or as a server).