
Mastering Vim
Build a software development environment with Vim and Neovim
Ruslan Osipov
- 330 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Mastering Vim
Build a software development environment with Vim and Neovim
Ruslan Osipov
About This Book
Mastering Vim, reviewed by Bram Moolenaar, the creator of Vim, covers usage of Vim and Neovim, showcases relevant plugins, and teaches Vimscript
Key Features
- Expert Vim and Vimscript techniques to work with Python and other development environment
- Accomplish end-to-end software development tasks with Neovim and Vim plugins
- Understand best practices for various facets of projects like version control, building, and testing
Book Description
Vim is a ubiquitous text editor that can be used for all programming languages. It has an extensive plugin system and integrates with many tools. Vim offers an extensible and customizable development environment for programmers, making it one of the most popular text editors in the world.
Mastering Vim begins with explaining how the Vim editor will help you build applications efficiently. With the fundamentals of Vim, you will be taken through the Vim philosophy. As you make your way through the chapters, you will learn about advanced movement, text operations, and how Vim can be used as a Python (or any other language for that matter) IDE. The book will then cover essential tasks, such as refactoring, debugging, building, testing, and working with a version control system, as well as plugin configuration and management. In the concluding chapters, you will be introduced to additional mindset guidelines, learn to personalize your Vim experience, and go above and beyond with Vimscript.
By the end of this book, you will be sufficiently confident to make Vim (or its fork, Neovim) your first choice when writing applications in Python and other programming languages.
What you will learn
- Get the most recent Vim, GVim, and Neovim versions installed
- Become efficient at navigating and editing text
- Uncover niche Vim plugins and pick the best ones
- Discover multiple ways of organizing plugins
- Explore and tailor Vim UI to fit your needs
- Organize and maintain Vim configuration across environments
- Write scripts to complement your workflow using Vimscript
Who this book is for
Mastering Vim is written for beginner, intermediate, and expert developers.The book will teach you to effectively embed Vim in your daily workflow. No prior experience with Python or Vim is required.
Frequently asked questions
Information
Transcending the Mundane with Vimscript
- The basic syntax, from declaring variables to using lambda expressions
- Style guides, and how to keep sane when developing in Vimscript
- A sample plugin from start to finishāfrom the first line to publishing it online
Technical requirements
Why Vimscript?
How to execute Vimscript
:source <filename>
:so %
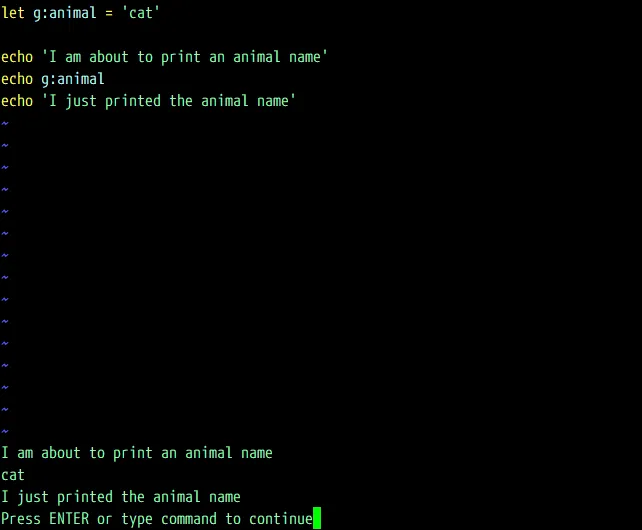
:echo g:animal
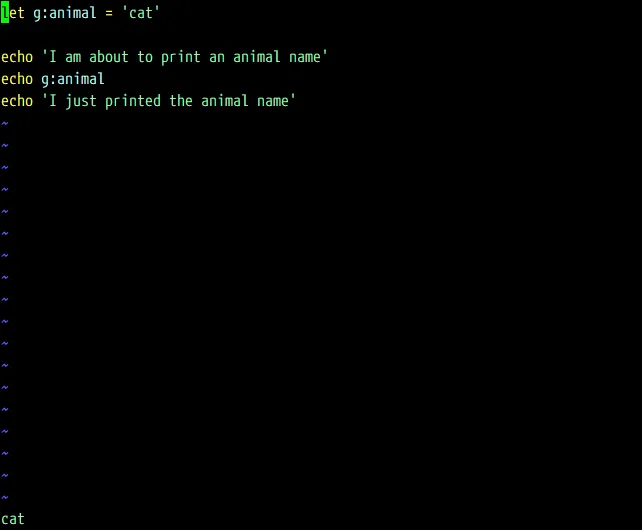
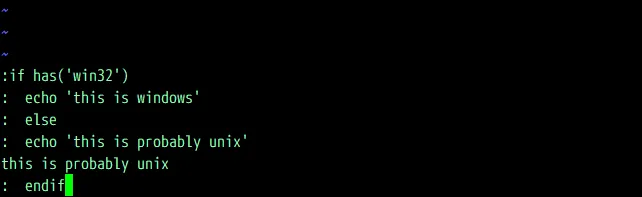
Learning the syntax
Setting variables
set background=dark
let animal_name = 'Miss Cattington'
let is_cat = 1
let g:animal_name = 'Miss Cattington'
let w:is_cat=1
- g: global scope (default if scope is not specified)
- v: global defined by Vim
- l: local scope (default within a function if scope is not specified)
- b: current buffer
- w: current window
- t: current tab
- s: local to a :source'd Vim script
- a: function argument
let @a = 'cats are weird'