
Hands-On Software Architecture with Golang
Design and architect highly scalable and robust applications using Go
Jyotiswarup Raiturkar
- 500 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Hands-On Software Architecture with Golang
Design and architect highly scalable and robust applications using Go
Jyotiswarup Raiturkar
About This Book
Understand the principles of software architecture with coverage on SOA, distributed and messaging systems, and database modeling
Key Features
- Gain knowledge of architectural approaches on SOA and microservices for architectural decisions
- Explore different architectural patterns for building distributed applications
- Migrate applications written in Java or Python to the Go language
Book Description
Building software requires careful planning and architectural considerations; Golang was developed with a fresh perspective on building next-generation applications on the cloud with distributed and concurrent computing concerns.
Hands-On Software Architecture with Golang starts with a brief introduction to architectural elements, Go, and a case study to demonstrate architectural principles. You'll then move on to look at code-level aspects such as modularity, class design, and constructs specific to Golang and implementation of design patterns. As you make your way through the chapters, you'll explore the core objectives of architecture such as effectively managing complexity, scalability, and reliability of software systems. You'll also work through creating distributed systems and their communication before moving on to modeling and scaling of data. In the concluding chapters, you'll learn to deploy architectures and plan the migration of applications from other languages.
By the end of this book, you will have gained insight into various design and architectural patterns, which will enable you to create robust, scalable architecture using Golang.
What you will learn
- Understand architectural paradigms and deep dive into Microservices
- Design parallelism/concurrency patterns and learn object-oriented design patterns in Go
- Explore API-driven systems architecture with introduction to REST and GraphQL standards
- Build event-driven architectures and make your architectures anti-fragile
- Engineer scalability and learn how to migrate to Go from other languages
- Get to grips with deployment considerations with CICD pipeline, cloud deployments, and so on
- Build an end-to-end e-commerce (travel) application backend in Go
Who this book is for
Hands-On Software Architecture with Golang is for software developers, architects, and CTOs looking to use Go in their software architecture to build enterprise-grade applications. Programming knowledge of Golang is assumed.
Frequently asked questions
Information
Modeling Data
- Entity-relationship modeling
- Engineering various consistency guarantees
- Relational data modeling and a hands-on deep-dive into MySQL
- Key value stores and a hands-on deep-dive into Redis
- Columnar stores and a hands-on deep-dive into Cassandra
- Patterns for scaling data stores
Entities and relationships
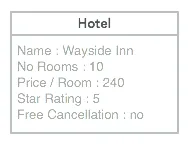

- Generalization: Formation of entity hierarchies to delineate various related entities
- Normalization: Removing redundancy in the modeled entities (we'll learn about this in detail in the Relational model section)
- Denormalization: Figuring out that redundancy is required and can help in increasing performance in certain use cases (covered in much more detail in the Scaling data section)
- Constraints/business rules: Governance on what values entity attributes can take and the relationships between entities
- Object relational mapper: Mapping of objects in the computation space with the entities persisted in the storage system
Consistency guarantees
ACID (Atomicity, Consistency, Isolation, Durability)
Atomicity
amountToTransfer:= 100 beginTransaction() srcValue:= getAccountBalance('abc') srcValue:= srcValue - amountToTransfer dstValue:= getAccountBalance('xyz') dstValue:= dstValue + amountToTransfer commitTransaction()