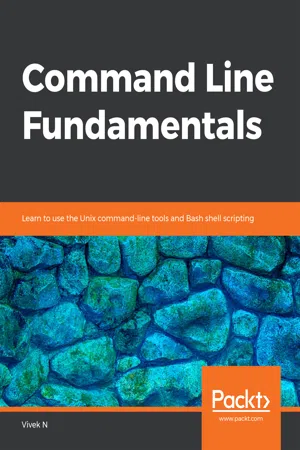
Command Line Fundamentals
Learn to use the Unix command-line tools and Bash shell scripting
Vivek N
- 314 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Command Line Fundamentals
Learn to use the Unix command-line tools and Bash shell scripting
Vivek N
About This Book
Master shell basics and Unix tools and discover easy commands to perform complex tasks with speed
Key Features
- Learn why the Bash shell is widely used on Linux and iOS
- Explore advanced shell concepts, such as pipes and redirection
- Understand how to use Unix command-line tools as building blocks for different tasks
Book Description
The most basic interface to a computerāthe command lineāremains the most flexible and powerful way of processing data and performing and automating various day-to-day tasks.
Command Line Fundamentals begins by exploring the basics, and then focuses on the most common tool, the Bash shell (which is standard on all Linux and iOS systems). As you make your way through the book, you'll explore the traditional Unix command-line programs as implemented by the GNU project. You'll also learn to use redirection and pipelines to assemble these programs to solve complex problems.
By the end of this book, you'll have explored the basics of shell scripting, allowing you to easily and quickly automate tasks.
What you will learn
- Use the Bash shell to run commands
- Utilize basic Unix utilities such as cat, tr, sort, and uniq
- Explore shell wildcards to manage groups of files
- Apply useful keyboard shortcuts in shell
- Employ redirection and pipes to process data
- Write both basic and advanced shell scripts to automate tasks
Who this book is for
Command Line Fundamentals is for programmers who use GUIs but want to understand how to use the command line to complete tasks faster.
Frequently asked questions
Information
Shell Scripting
Learning Objectives
- Use looping and conditional constructs in the shell
- Use shell functions
- Perform line-oriented processing using the shell language
- Write shell scripts
- Automate data processing tasks with scripts
Introduction
Conditionals and Loops
Note
Conditional Expressions
- -e FILE: Returns true if FILE exists either as a file or a directory. For instance, let's create a file called test and check whether it exists using this operator:robin ~ $ touch testrobin ~ $ [[ -e test ]] && echo File or directory existsFile or directory existsWe can also use this to check for files that do not exist, as follows:robin ~ $ [[ -e none ]] || echo File or directory missingFile or directory missing
- -f FILE: Returns true if FILE exists. For instance, look at the following snippet:robin ~ $ touch testrobin ~ $ [[ -f test ]] && echo test exists and is a filetest exists and is a file
- -d DIR: Returns true if the directory DIR exists. For instance, look at the following snippet:robin ~ $ mkdir dirrobin ~ $ [[ -d dir ]] && echo Dir existsDir existsrobin ~ $ [[ -d dir1 ]] || echo Dir missingDir missing
- -z STRING: Returns true if the string is empty. Look at the following example:robin ~ $ [[ -z '' ]] && echo EMPTYEMPTY
- -n STRING: Returns true if the string is not empty (its length is not zero), as shown here:robin ~ $ [[ -n 'SOMETHING' ]] && echo NOT EMPTYNOT EMPTY
- STRING1 = STRING2 or STRING1 == STRING2: Returns true if the two strings are identical. Let's understand this better with an example. In the following snippet, we have defined three variables with strings, as follows:robin ~ $ A=APPLErobin ~ $ B=HELLOrobin ~ $ C=HELLOWe can use the preceding operator to compare any two strings, as shown here:robin ~ $ [[ "$B" = "$C" ]] && echo SameSame
- STRING1 != STRING2: Returns true if the strings are not identical. For instance, for the preceding example, the following will be true:robin ~ $ [[ "$A" != "$C" ]] && echo DifferentDifferent
- STRING1 < STRING2: Returns true if the first string is lexicographically lower. Look at the following example:robin ~ $ [[ "$A" < "$C" ]] && echo LessLess
- STRING1 > STRING2: Returns true if the first string is lexicographically higher, as shown in the following example:robin ~ $ [[ "$C" > "$A" ]] && echo GreaterGreater
Note
Remember to always use double quotes for string matching. Whether you use a variable or a literal string as either operand, this is recommended since strings can contain spaces, and other characters. We cannot use single quotes since the variables will not expand to their values.
- STRING = PATTERN or STRING == PATTERN: Returns true if the string matches the glob PATTERN.
- STRING != PATTERN: Returns true if the string does not match the glob PATTERN.