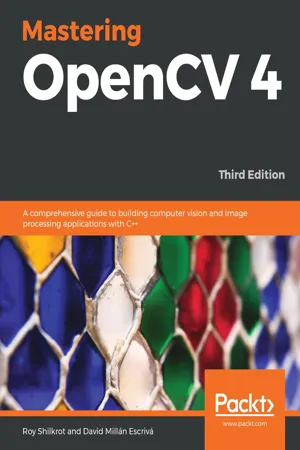
Mastering OpenCV 4
A comprehensive guide to building computer vision and image processing applications with C++, 3rd Edition
Roy Shilkrot, David Millán Escrivá
- 280 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Mastering OpenCV 4
A comprehensive guide to building computer vision and image processing applications with C++, 3rd Edition
Roy Shilkrot, David Millán Escrivá
About This Book
Work on practical computer vision projects covering advanced object detector techniques and modern deep learning and machine learning algorithms
Key Features
- Learn about the new features that help unlock the full potential of OpenCV 4
- Build face detection applications with a cascade classifier using face landmarks
- Create an optical character recognition (OCR) model using deep learning and convolutional neural networks
Book Description
Mastering OpenCV, now in its third edition, targets computer vision engineers taking their first steps toward mastering OpenCV. Keeping the mathematical formulations to a solid but bare minimum, the book delivers complete projects from ideation to running code, targeting current hot topics in computer vision such as face recognition, landmark detection and pose estimation, and number recognition with deep convolutional networks.
You'll learn from experienced OpenCV experts how to implement computer vision products and projects both in academia and industry in a comfortable package. You'll get acquainted with API functionality and gain insights into design choices in a complete computer vision project. You'll also go beyond the basics of computer vision to implement solutions for complex image processing projects.
By the end of the book, you will have created various working prototypes with the help of projects in the book and be well versed with the new features of OpenCV4.
What you will learn
- Build real-world computer vision problems with working OpenCV code samples
- Uncover best practices in engineering and maintaining OpenCV projects
- Explore algorithmic design approaches for complex computer vision tasks
- Work with OpenCV's most updated API (v4.0.0) through projects
- Understand 3D scene reconstruction and Structure from Motion (SfM)
- Study camera calibration and overlay AR using the ArUco Module
Who this book is for
This book is for those who have a basic knowledge of OpenCV and are competent C++ programmers. You need to have an understanding of some of the more theoretical/mathematical concepts, as we move quite quickly throughout the book.
Frequently asked questions
Information
Face Detection and Recognition with the DNN Module
- Face detection with different methods
- Face preprocessing
- Training a machine learning algorithm from collected faces
- Face recognition
- Finishing touches
Introduction to face detection and face recognition
- Face detection: This is the process of locating a face region in an image (the large rectangle near the center of the following screenshot). This step does not care who the person is, just that it is a human face.
- Face preprocessing: This is the process of adjusting the face image to look clearer and similar to other faces (a small grayscale face in the top center of the following screenshot).
- Collecting and learning faces: This is a process of saving many preprocessed faces (for each person that should be recognized), and then learning how to recognize them.
- Face recognition: This is the process that checks which of the collected people are most similar to the face in the camera (a small rectangle in the top right of the following screenshot).

Face detection
Implementing face detection using OpenCV cascade classifiers
Type of cascade classifier | XML filename |
Face detector (default) | haarcascade_frontalface_default.xml |
Face detector (fast Haar) | haarcascade_frontalface_alt2.xml |
Face detector (fast LBP) | lbpcascade_frontalface.xml |
Profile (side-looking) face detector | haarcascade_profileface.xml |
Eye detector (separate for left and right) | haarcascade_lefteye_2splits.xml |
Mouth detector | haarcascade_mcs_mouth.x... |