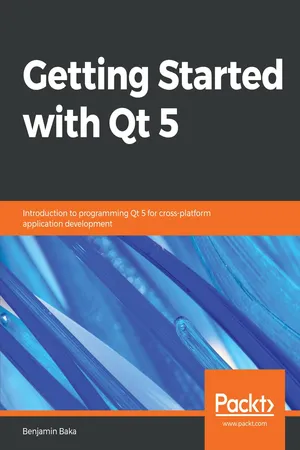
Getting Started with Qt 5
Introduction to programming Qt 5 for cross-platform application development
Benjamin Baka
- 136 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Getting Started with Qt 5
Introduction to programming Qt 5 for cross-platform application development
Benjamin Baka
About This Book
Begin writing graphical user interface(GUI) applications for building human machine interfaces with a clear understanding of key concepts of the Qt framework
Key Features
- Learn how to write, assemble, and build Qt application from the command line
- Understand key concepts like Signals and Slots in Qt
- Best practices and effective techniques for designing graphical user interfaces using Qt 5
Book Description
Qt is a cross-platform application framework and widget toolkit that is used to create GUI applications that can run on different hardware and operating systems. The main aim of this book is to introduce Qt to the reader. Through the use of simple examples, we will walk you through building blocks without focusing too much on theory.
Qt is a popular tool that can be used for building a variety of applications, such as web browsers, media players such as VLC, and Adobe Photoshop. Following Qt installation and setup, the book dives straight into helping you create your first application.
You will be introduced to Widgets, Qt's interface building block, and the many varieties that are available for creating GUIs. Next, Qt's core concept of signals and slots are well illustrated with sufficient examples. The book further teaches you how to create custom widgets, signals and slots, and how to communicate useful information via dialog boxes. To cap everything off, you will be taken through writing applications that can connect to databases in order to persist data.
By the end of the book, you should be well equipped to start creating your own Qt applications and confident enough to pick up more advanced Qt techniques and materials to hone your skills.
What you will learn
- Set up and configure your machine to begin developing Qt applications
- Discover different widgets and layouts for constructing UIs
- Understand the key concept of signals and slots
- Understand how signals and slots help animate a GUI
- Explore how to create customized widgets along with signals and slots
- Understand how to subclass and create a custom windows application
- Understand how to write applications that can talk to databases.
Who this book is for
Anyone trying to start development of graphical user interface application will find this book useful. One does not need prior exposure to other toolkits to understand this book. In order to learn from this book you should have basic knowledge of C++ and a good grasp of Object Oriented Programming. Familiarity with GNU/Linux will be very useful though it's not a mandatory skill.
Frequently asked questions
Information
Implementing Windows and Dialog
- Understand how to subclass and create a custom window application
- Add a menu bar to a window
- Add a toolbar to a window
- Use the various dialog (boxes) to communicate information to the user
Creating a custom window
#include <QApplication>
#include <QMainWindow>
#include <QLabel>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QMainWindow mainWindow;
mainWindow.show();
return a.exec();
}
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QLabel>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow();
};
#endif
public:
MainWindow();
#include "mainwindow.h"
MainWindow::MainWindow()
{
setWindowTitle("Main Window");
resize(400, 700);
QLabel *mainLabel = new QLabel("Main Widget");
setCentralWidget(mainLabel);
mainLabel->setAlignment(Qt::AlignCenter);
}
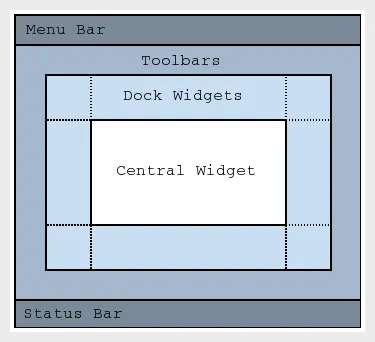
#include <QApplication>
#include "mainwindow.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MainWindow mainwindow;
mainwindow.show();
return app.exec();
}
% qmake -project
% qmake
% make
HEADERS += mainwindow.h
SOURCES += main.cpp mainwindow.cpp
% ./classSimpleWindow