
Hands-On Network Programming with C# and .NET Core
Build robust network applications with C#Â and .NET Core
Sean Burns
- 488 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Hands-On Network Programming with C# and .NET Core
Build robust network applications with C#Â and .NET Core
Sean Burns
About This Book
A comprehensive guide to understanding network architecture, communication protocols, and network analysis to build secure applications compatible with the latest versions of C# 8 and.NET Core 3.0
Key Features
- Explore various network architectures that make distributed programming possible
- Learn how to make reliable software by writing secure interactions between clients and servers
- Use.NET Core for network device automation, DevOps, and software-defined networking
Book Description
The C# language and the.NET Core application framework provide the tools and patterns required to make the discipline of network programming as intuitive and enjoyable as any other aspect of C# programming. With the help of this book, you will discover how the C# language and the.NET Core framework make this possible.
The book begins by introducing the core concepts of network programming, and what distinguishes this field of programming from other disciplines. After this, you will gain insights into concepts such as transport protocols, sockets and ports, and remote data streams, which will provide you with a holistic understanding of how network software fits into larger distributed systems. The book will also explore the intricacies of how network software is implemented in a more explicit context, by covering sockets, connection strategies such as Transmission Control Protocol (TCP) and User Datagram Protocol (UDP), asynchronous processing, and threads. You will then be able to work through code examples for TCP servers, web APIs served over HTTP, and a Secure Shell (SSH) client.
By the end of this book, you will have a good understanding of the Open Systems Interconnection (OSI) network stack, the various communication protocols for that stack, and the skills that are essential to implement those protocols using the C# programming language and the.NET Core framework.
What you will learn
- Understand the breadth of C#'s network programming utility classes
- Utilize network-layer architecture and organizational strategies
- Implement various communication and transport protocols within C#
- Discover hands-on examples of distributed application development
- Gain hands-on experience with asynchronous socket programming and streams
- Learn how C# and the.NET Core runtime interact with a hosting network
- Understand a full suite of network programming tools and features
Who this book is for
If you're a.NET developer or a system administrator with.NET experience and are looking to get started with network programming, then this book is for you. Basic knowledge of C# and.NET is assumed, in addition to a basic understanding of common web protocols and some high-level distributed system designs.
Frequently asked questions
Information
Section 1: Foundations of Network Architecture
Chapter 2, DNS and Resource Location
Chapter 3, Communication Protocols
Chapter 4, Packets and Streams
Networks in a Nutshell
- The unique challenges of distributing computational or data resources over a network, and how those challenges manifest in software
- The different components of a network, and how those components can be arranged to achieve different goals
- The impact of the variability of devices, latency, instability, and standardization of networks on the complexity of applications written for network use
- Common concepts, terms, and data structures used for network programming, and how those concepts are exposed by .NET Core
- Understanding the scope of applications that are made possible by networked architectures, and the importance of developing skills in network programming to enable those kinds of applications
Technical requirements
Expanding the scope of software â distributed systems and the challenges they introduce
What is a network?
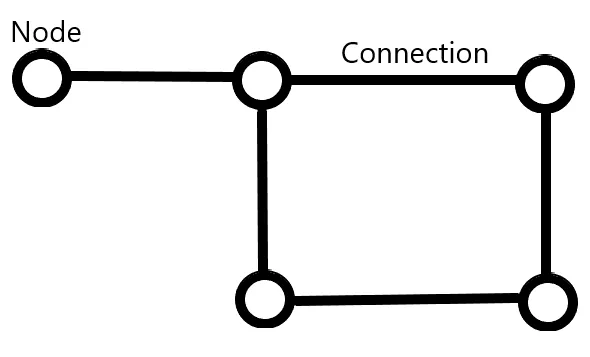
A computer network is, for our purposes, an arbitrarily large set of computational or navigational devices, connected by channels of communication across which computational resources can be reliably sent, received, forwarded, or processed.