
Hands-On Network Programming with C
Learn socket programming in C and write secure and optimized network code
Lewis Van Winkle
- 478 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
Hands-On Network Programming with C
Learn socket programming in C and write secure and optimized network code
Lewis Van Winkle
About This Book
A comprehensive guide to programming with network sockets, implementing internet protocols, designing IoT devices, and much more with C
Key Features
- Apply your C and C++ programming skills to build powerful network applications
- Get to grips with a variety of network protocols that allow you to load web pages, send emails, and do much more
- Write portable network code for Windows, Linux, and macOS
Book Description
Network programming enables processes to communicate with each other over a computer network, but it is a complex task that requires programming with multiple libraries and protocols. With its support for third-party libraries and structured documentation, C is an ideal language to write network programs.
Complete with step-by-step explanations of essential concepts and practical examples, this C network programming book begins with the fundamentals of Internet Protocol, TCP, and UDP. You'll explore client-server and peer-to-peer models for information sharing and connectivity with remote computers. The book will also cover HTTP and HTTPS for communicating between your browser and website, and delve into hostname resolution with DNS, which is crucial to the functioning of the modern web. As you advance, you'll gain insights into asynchronous socket programming and streams, and explore debugging and error handling. Finally, you'll study network monitoring and implement security best practices.
By the end of this book, you'll have experience of working with client-server applications and be able to implement new network programs in C.
The code in this book is compatible with the older C99 version as well as the latest C18 and C++17 standards. You'll work with robust, reliable, and secure code that is portable across operating systems, including Winsock sockets for Windows and POSIX sockets for Linux and macOS.
What you will learn
- Uncover cross-platform socket programming APIs
- Implement techniques for supporting IPv4 and IPv6
- Understand how TCP and UDP connections work over IP
- Discover how hostname resolution and DNS work
- Interface with web APIs using HTTP and HTTPS
- Explore Simple Mail Transfer Protocol (SMTP) for electronic mail transmission
- Apply network programming to the Internet of Things (IoT)
Who this book is for
If you're a developer or a system administrator who wants to get started with network programming, this book is for you. Basic knowledge of C programming is assumed.
Frequently asked questions
Information
Section 1 - Getting Started with Network Programming
Introducing Networks and Protocols
- Network programming and C
- OSI layer model
- TCP/IP reference model
- The Internet Protocol
- IPv4 addresses and IPv6 addresses
- Domain names
- Internet protocol routing
- Network address translation
- The client-server paradigm
- Listing your IP addresses programmatically from C
Technical requirements
git clone https://github.com/codeplea/Hands-On-Network-Programming-with-C
cd Hands-On-Network-Programming-with-C/chap01
gcc win_list.c -o win_list.exe -liphlpapi -lws2_32
win_list
gcc unix_list.c -o unix_list
./unix_list
The internet and C
OSI layer model
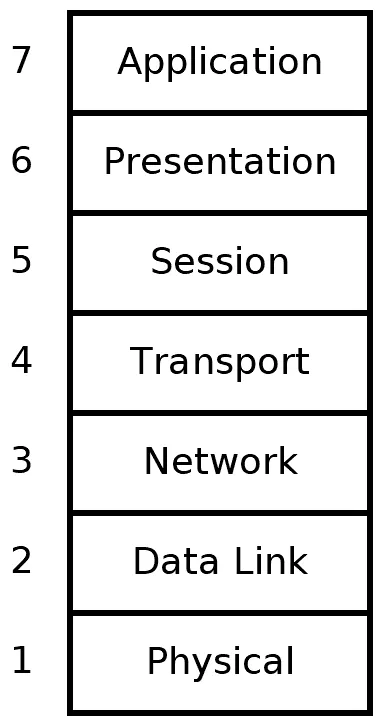
- Physical (1): This is the level of physical communication in the real world. At this level, we have specifications for things such as the voltage levels on an Ethernet cable, what each pin on a connector is for, the radio frequency of Wi-Fi, and the light flashes over an optic fiber.
- Data Link (2): This level builds on the physical layer. It deals with protocols for directly communicating between two nodes. It defines how a direct message between nodes starts and ends (framing), error detection and correction, and flow control.
- Network layer (3): The network layer provides the methods to transmit data sequences (called packets) between nodes in different networks. It provides methods to route packets from one node to another (without a direct physical connection) by transferring through many intermediate nodes. This is the layer that the Internet Protocol is defined on, which we will go into in some depth later.
- Transport layer (4): At this layer, we have methods to reliably deliver variable length data between hosts. These methods deal with splitting up data, recombining it, ensuring data arrives in ord...