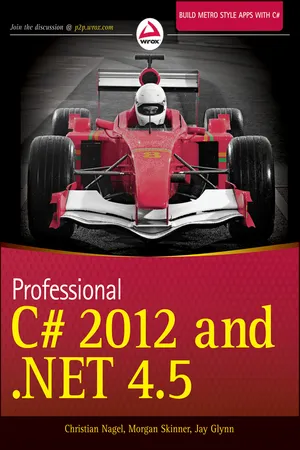
eBook - ePub
Professional C# 2012 and .NET 4.5
Christian Nagel, Bill Evjen, Jay Glynn, Karli Watson, Morgan Skinner
This is a test
- English
- ePUB (handyfreundlich)
- Über iOS und Android verfügbar
eBook - ePub
Professional C# 2012 and .NET 4.5
Christian Nagel, Bill Evjen, Jay Glynn, Karli Watson, Morgan Skinner
Angaben zum Buch
Buchvorschau
Inhaltsverzeichnis
Quellenangaben
Über dieses Buch
Intermediate to advanced technique coverage, updated for C# 2012 and.NET 4.5
This guide is geared towards experienced programmers looking to update and enhance their skills in writing Windows applications, web apps, and Metro apps with C# and.NET 4.5. Packed with information about intermediate and advanced features, this book includes everything professional developers need to know about C# and putting it to work.
- Covers challenging.NET features including Language Integrated Query (LINQ), LINQ to SQL, LINQ to XML, WCF, WPF, Workflow, and Generics
- Puts the new Async keyword to work and features refreshers on.NET architecture, objects, types, inheritance, arrays, operators, casts, delegates, events, strings, regular expressions, collections, and memory management
- Explores new options and interfaces presented by Windows 8 development, WinRT, and Metro style apps
- Includes traditional Windows forms programming, ASP.NET web programming with C#, and working in Visual Studio 2012 with C#
Professional C# 2012 and.NET 4.5 is a comprehensive guide for experienced programmers wanting to maximize these technologies.
Häufig gestellte Fragen
Wie kann ich mein Abo kündigen?
Gehe einfach zum Kontobereich in den Einstellungen und klicke auf „Abo kündigen“ – ganz einfach. Nachdem du gekündigt hast, bleibt deine Mitgliedschaft für den verbleibenden Abozeitraum, den du bereits bezahlt hast, aktiv. Mehr Informationen hier.
(Wie) Kann ich Bücher herunterladen?
Derzeit stehen all unsere auf Mobilgeräte reagierenden ePub-Bücher zum Download über die App zur Verfügung. Die meisten unserer PDFs stehen ebenfalls zum Download bereit; wir arbeiten daran, auch die übrigen PDFs zum Download anzubieten, bei denen dies aktuell noch nicht möglich ist. Weitere Informationen hier.
Welcher Unterschied besteht bei den Preisen zwischen den Aboplänen?
Mit beiden Aboplänen erhältst du vollen Zugang zur Bibliothek und allen Funktionen von Perlego. Die einzigen Unterschiede bestehen im Preis und dem Abozeitraum: Mit dem Jahresabo sparst du auf 12 Monate gerechnet im Vergleich zum Monatsabo rund 30 %.
Was ist Perlego?
Wir sind ein Online-Abodienst für Lehrbücher, bei dem du für weniger als den Preis eines einzelnen Buches pro Monat Zugang zu einer ganzen Online-Bibliothek erhältst. Mit über 1 Million Büchern zu über 1.000 verschiedenen Themen haben wir bestimmt alles, was du brauchst! Weitere Informationen hier.
Unterstützt Perlego Text-zu-Sprache?
Achte auf das Symbol zum Vorlesen in deinem nächsten Buch, um zu sehen, ob du es dir auch anhören kannst. Bei diesem Tool wird dir Text laut vorgelesen, wobei der Text beim Vorlesen auch grafisch hervorgehoben wird. Du kannst das Vorlesen jederzeit anhalten, beschleunigen und verlangsamen. Weitere Informationen hier.
Ist Professional C# 2012 and .NET 4.5 als Online-PDF/ePub verfügbar?
Ja, du hast Zugang zu Professional C# 2012 and .NET 4.5 von Christian Nagel, Bill Evjen, Jay Glynn, Karli Watson, Morgan Skinner im PDF- und/oder ePub-Format sowie zu anderen beliebten Büchern aus Informatique & Programmation en C#. Aus unserem Katalog stehen dir über 1 Million Bücher zur Verfügung.
Information
Part I
The C# Language
- CHAPTER 1: .NET Architecture
- CHAPTER 2: Core C#
- CHAPTER 3: Objects and Types
- CHAPTER 4: Inheritance
- CHAPTER 5: Generics
- CHAPTER 6: Arrays and Tuples
- CHAPTER 7: Operators and Casts
- CHAPTER 8: Delegates, Lambdas, and Events
- CHAPTER 9: Strings and Regular Expressions
- CHAPTER 10: Collections
- CHAPTER 11: Language Integrated Query
- CHAPTER 12: Dynamic Language Extensions
- CHAPTER 13: Asynchronous Programming
- CHAPTER 14: Memory Management and Pointers
- CHAPTER 15: Reflection
- CHAPTER 16: Errors and Exceptions
Chapter 1
.NET Architecture
WHAT’S IN THIS CHAPTER?
- Compiling and running code that targets .NET
- Advantages of Microsoft Intermediate Language (MSIL)
- Value and reference types
- Data typing
- Understanding error handling and attributes
- Assemblies, .NET base classes, and namespaces
WROX.COM CODE DOWNLOADS FOR THIS CHAPTER
There are no code downloads for this chapter.
THE RELATIONSHIP OF C# TO .NET
This book emphasizes that the C# language must be considered in parallel with the .NET Framework, rather than viewed in isolation. The C# compiler specifically targets .NET, which means that all code written in C# always runs within the .NET Framework. This has two important consequences for the C# language:
1. The architecture and methodologies of C# reflect the underlying methodologies of .NET.
2. In many cases, specific language features of C# actually depend on features of .NET or of the .NET base classes.
Because of this dependence, you must gain some understanding of the architecture and methodology of .NET before you begin C# programming, which is the purpose of this chapter.
C# is a programming language newly designed for .NET. and is significant in two respects:
- It is specifically designed and targeted for use with Microsoft’s .NET Framework (a feature-rich platform for the development, deployment, and execution of distributed applications).
- It is a language based on the modern object-oriented design methodology, and when designing it Microsoft learned from the experience of all the other similar languages that have been around since object-oriented principles came to prominence 20 years ago.
C# is a language in its own right. Although it is designed to generate code that targets the .NET environment, it is not part of .NET. Some features are supported by .NET but not by C#, and you might be surprised to learn that some features of the C# language are not supported by .NET (for example, some instances of operator overloading).
However, because the C# language is intended for use with .NET, you must understand this Framework if you want to develop applications in C# effectively. Therefore, this chapter takes some time to peek underneath the surface of .NET.
THE COMMON LANGUAGE RUNTIME
Central to the .NET Framework is its runtime execution environment, known as the Common Language Runtime (CLR) or the .NET runtime. Code running under the control of the CLR is often termed managed code.
However, before it can be executed by the CLR, any source code that you develop (in C# or some other language) needs to be compiled. Compilation occurs in two steps in .NET:
1. Compilation of source code to Microsoft Intermediate Language (IL).
2. Compilation of IL to platform-specific code by the CLR.
This two-stage compilation process is important because the existence of the Microsoft Intermediate Language is the key to providing many of the benefits of .NET.
IL shares with Java byte code the idea that it is a low-level language with a simple syntax (based on numeric codes rather than text), which can be quickly translated into native machine code. Having this well-defined universal syntax for code has significant advantages: platform independence, performance improvement, and language interoperability.
Platform Independence
First, platform independence means that the same file containing byte code instructions can be placed on any platform; at runtime, the final stage of compilation can then be easily accomplished so that the code can run on that particular platform. In other words, by compiling to IL you obtain platform independence for .NET in much the same way as compiling to Java byte code gives Java platform independence.
The platform independence of .NET is only theoretical at present because, at the time of writing, a complete implementation of .NET is available only for Windows. However, a partial, cross-platform implementation is available (see, for example, the Mono project, an effort to create an open source implementation of .NET, at www.go-mono.com).
Performance Improvement
Although previously compared to Java, IL is actually a bit more ambitious than Java byte code. IL is always Just-in-Time compiled (known as JIT compilation), whereas Java byte code was often interpreted. One of the disadvantages of Java was that, on execution, the process to translate from Java byte code to native executable resulted in a loss of performance (with the exception of more recent cases in which Java is JIT compiled on certain platforms).
Instead of compiling the entire application at one time (which could lead to a slow startup time), the JIT compiler simply compiles each portion of code as it is called (just in time). When code has been compiled once, the resultant native executable is stored until the application exits so that it does not need to be recompiled the next time that portion of code is run. Microsoft argues that this process is more efficient than compiling the entire application code at the start because of the likelihood that large portions of any application code will not actually be executed in any given run. Using the JIT compiler, such code can never be compiled.
This explains why you can expect that execution of managed IL code will be almost as fast as executing native machine code. What it does not explain is why Microsoft expects that you get a performance improvement. The reason given for this is that because the final stage of compilation takes place at runtime, the JIT compiler knows exactly what processor type the program runs on. This means that it can optimize the final executable code to take advantage of any features or particular machine code instructions offered by that particular processor.
Traditional compilers optimize the code, but they can perform optimizations that are only independent of the particular processor that the code runs on. This is because traditional compilers compile to native executable code before the software is shipped. This means that the compiler does not know what type of processor the code runs on beyond basic generalities, such as that it is an x86-compatible processor or an Alpha processor.
Language Interoperability
The use of IL not only enables platform independence, but it also facilitates language interoperability. Simply put, you can compile to IL from one language, and this compiled code should then be interoperable with code that has been compiled to IL from another language.
You are probably now wondering which languages aside from C# are interoperable with .NET. The following sections briefly discuss how some of the other common languages fit into .NET.
Visual Basic 2012
Visual Basic .NET 2002 underwent a complete revamp from Visual Basic 6 to bring it up to date with the first version of the .NET Framework. The Visual Basic language had dramatically evolved from VB6, which this meant that VB6 was not a suitable language to run .NET programs. For example, VB6 is heavily integrated into Component Object Model (COM) and works by exposing only event handlers as source code to the developer — most of the background code is not available as source code. Not only that, it does not support implementation inheritance, and the st...
Inhaltsverzeichnis
Zitierstile für Professional C# 2012 and .NET 4.5
APA 6 Citation
Nagel, C., Evjen, B., Glynn, J., Watson, K., & Skinner, M. (2012). Professional C# 2012 and .NET 4.5 (1st ed.). Wiley. Retrieved from https://www.perlego.com/book/1003889/professional-c-2012-and-net-45-pdf (Original work published 2012)
Chicago Citation
Nagel, Christian, Bill Evjen, Jay Glynn, Karli Watson, and Morgan Skinner. (2012) 2012. Professional C# 2012 and .NET 4.5. 1st ed. Wiley. https://www.perlego.com/book/1003889/professional-c-2012-and-net-45-pdf.
Harvard Citation
Nagel, C. et al. (2012) Professional C# 2012 and .NET 4.5. 1st edn. Wiley. Available at: https://www.perlego.com/book/1003889/professional-c-2012-and-net-45-pdf (Accessed: 14 October 2022).
MLA 7 Citation
Nagel, Christian et al. Professional C# 2012 and .NET 4.5. 1st ed. Wiley, 2012. Web. 14 Oct. 2022.