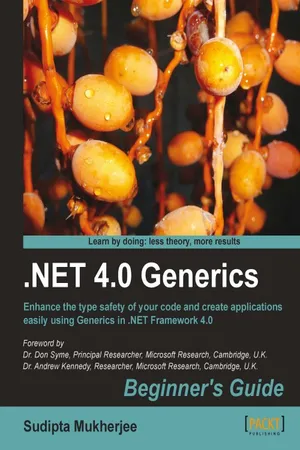
.NET 4.0 Generics Beginner's Guide
Sudipta Mukherjee
- 396 Seiten
- English
- ePUB (handyfreundlich)
- Ăber iOS und Android verfĂŒgbar
.NET 4.0 Generics Beginner's Guide
Sudipta Mukherjee
Ăber dieses Buch
In Detail
Generics were added as part of .NET Framework 2.0 in November 2005. Although similar to generics in Java, .NET generics do not apply type erasure but every object has unique representation at run-time. There is no performance hit from runtime casts and boxing conversions, which are normally expensive..NET offers type-safe versions of every classical data structure and some hybrid ones.
This book will show you everything you need to start writing type-safe applications using generic data structures available in Generics API. You will also see how you can use several collections for each task you perform. This book is full of practical examples, interesting applications, and comparisons between Generics and more traditional approaches. Finally, each container is bench marked on the basis of performance for a given task, so you know which one to use and when.
This book first covers the fundamental concepts such as type safety, Generic Methods, and Generic Containers. As the book progresses, you will learn how to join several generic containers to achieve your goals and query them efficiently using Linq. There are short exercises in every chapter to boost your knowledge.
The book also teaches you some best practices, and several patterns that are commonly available in generic code.
Some important generic algorithm definitions are present in Power Collection (an API created by Wintellect Inc.) that are missing from .NET framework. This book shows you how to use such algorithms seamlessly with other generic containers.
The book also discusses C5 collections. Java Programmers will find themselves at home with this API. This is the closest to JCF. Some very interesting problems are solved using generic containers from .NET framework, C5, and PowerCollection Algorithms - a clone of Google Set and Gender Genie for example!
The author has also created a website (http://www.consulttoday.com/genguide) for the book where you can find many useful tools, code snippets, and, applications, which are not the part of code-download section
Approach
This is a concise, practical guide that will help you learn Generics in .NET, with lots of real world and fun-to-build examples and clear explanations. It is packed with screenshots to aid your understanding of the process.
Who this book is for
This book is aimed at beginners in Generics. It assumes some working knowledge of C# , but it isn't mandatory.
The following would get the most use out of the book:
- Newbie C# developers struggling with Generics.
- Experienced C++ and Java Programmers who are migrating to C# and looking for an alternative to other generic frameworks like STL and JCF would find this book handy.
- Managers who want to know what Generics is and how to put it to good use.
- Architects will find the benchmarking extremely useful, because it's the first of its kind across a framework of several collections.