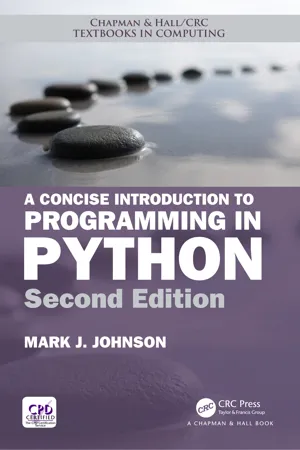
A Concise Introduction to Programming in Python
Mark J. Johnson
- 209 pagine
- English
- ePUB (disponibile sull'app)
- Disponibile su iOS e Android
A Concise Introduction to Programming in Python
Mark J. Johnson
Informazioni sul libro
A Concise Introduction to Programming in Python, Second Edition provides a hands-on and accessible introduction to writing software in Python, with no prior programming experience required.
The Second Edition was thoroughly reorganized and rewritten based on classroom experience to incorporate:
- A spiral approach, starting with turtle graphics, and then revisiting concepts in greater depth using numeric, textual, and image data
-
- Clear, concise explanations written for beginning students, emphasizing core principles
-
- A variety of accessible examples, focusing on key concepts
-
- Diagrams to help visualize new concepts
-
- New sections on recursion and exception handling, as well as an earlier introduction of lists, based on instructor feedback
The text offers sections designed for approximately one class period each, and proceeds gradually from procedural to object-oriented design. Examples, exercises, and projects are included from diverse application domains, including finance, biology, image processing, and textual analysis. It also includes a brief "How-To" sections that introduce optional topics students may be interested in exploring.
The text is written to be read, making it a good fit in flipped classrooms. Designed for either classroom use or self-study, all example programs and solutions to odd-numbered exercises (except for projects) are available at: http://www.central.edu/go/conciseintro/.
Domande frequenti
Informazioni
CHAPTER 1
Turtle Graphics
1.1 GETTING STARTED
www.python.org
. Follow the instructions given there to install the latest production version of Python 3 on your system. All examples in this text were written with Python 3.6.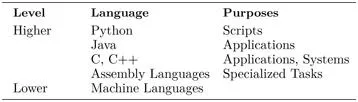
” prompt. The interpreter will translate and execute any legal Python code that is typed at this prompt. For example, if we enter the following statement: