
eBook - ePub
Fluid Engine Development
Doyub Kim
This is a test
Share book
- 300 pages
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Fluid Engine Development
Doyub Kim
Book details
Book preview
Table of contents
Citations
About This Book
From the splash of breaking waves to turbulent swirling smoke, the mathematical dynamics of fluids are varied and continue to be one of the most challenging aspects in animation. Fluid Engine Development demonstrates how to create a working fluid engine through the use of particles and grids, and even a combination of the two. Core algorithms are explained from a developer's perspective in a practical, approachable way that will not overwhelm readers. The Code Repository offers further opportunity for growth and discussion with continuously changing content and source codes. This book helps to serve as the ultimate guide to navigating complex fluid animation and development.
- Explains how to create a fluid simulation engine from scratch
- Offers an approach that is code-oriented rather than math-oriented, allowing readers to learn how fluid dynamics works with code, with downloadable code available
-
- Explores various kinds of simulation techniques for fluids using particles and grids
-
- Discusses practical issues such as data structure design and optimizations
-
- Covers core numerical tools including linear system and level set solvers
-
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is Fluid Engine Development an online PDF/ePUB?
Yes, you can access Fluid Engine Development by Doyub Kim in PDF and/or ePUB format, as well as other popular books in Computer Science & Computer Graphics. We have over one million books available in our catalogue for you to explore.
Information
1
Basics
This chapter covers the most fundamental topics that will frequently be referred throughout the book. Before anything else, a minimal fluid simulator will be introduced to bring a basic understanding of building a simulation engine. We will then start building up the foundation for mathematical and geometric operations that are commonly used in this book. This chapter will also introduce the core concept of computer-generated animations as well as its implementation, which will then evolve to the physics-based animation. Finally, the general process of simulating fluid flow will be introduced at the end of the chapter.
1.1 Hello, Fluid Simulator
In this section, we will implement the simplest fluid simulator in this book. This minimal example may not be fancy at all, but it will cover the key ideas of the fluid simulation engine end to end. It is self-contained and doesnât depend on any other libraries other than the standard C++ library. Although we havenât discussed anything about the simulation yet, this hello-world example will provide insight into how to approach to writing a fluid engine code. The only prerequisite for this is some knowledge on C++ and ability to run it on a command line tool.
The goal of the example is simple: to simulate two traveling waves in a one-dimensional (1D) world as shown in Figure 1.1. When a wave hits the end of the container, it will bounce back to the opposite direction.
1.1.1 Defining State
Before we start coding, letâs step back and imagine how we would describe the waves with code. At a given time, a wave is located at a certain position with its speed as illustrated in Figure 1.2. Also, as shown in the figure, the final shape can be constructed from the wave positions. Therefore, the state of a wave can be simply defined as a pair of the position and speed. Since we have two waves, we need two pairs of states. Extremely straightforward and nothing complicated.
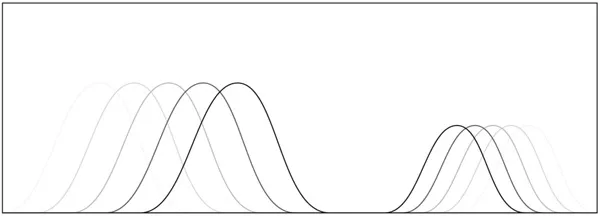
FIGURE 1.1
Simple 1D wave animation. Two different waves are moving back and forth.
Simple 1D wave animation. Two different waves are moving back and forth.
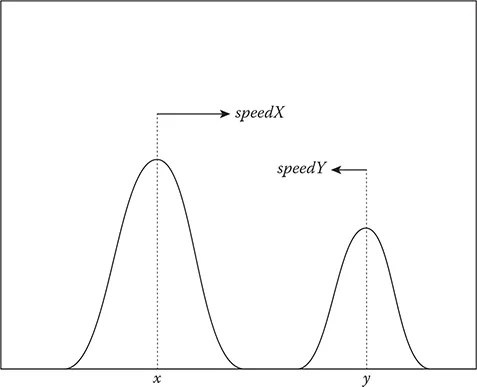
FIGURE 1.2
State of the two waves are described by their positions and speeds.
State of the two waves are described by their positions and speeds.
Now, itâs time to code. Consider the following:
1 #include <cstdio>
2
3 int main() {
4 double x = 0.0;
5 double y = 1.0;
6 double speedX = 1.0;
7 double speedY = -0.5;
8
9 return 0;
10 }
2
3 int main() {
4 double x = 0.0;
5 double y = 1.0;
6 double speedX = 1.0;
7 double speedY = -0.5;
8
9 return 0;
10 }
When naming the variables, we will use alphabet X to refer one of the waves and Y for the other one. As shown in the code, initial values assigned to those variables tells us that wave X starts from the left-most side (
double x = 0.0
) and travels to the right with 1.0 speed (double speedX = 1.0
). Similarly, wave Y starts from the right-most side (double y = 1.0
) and travels to the left (double speedY = -0.5
) with half of the magnitude of wave Xâs speed.Note that we just defined the âstatesâ of the simulation using four variables which are the most crucial steps when designing a simulation engine. In this particular example, the simulation state is simply the positions and velocities of the waves. But in more complex systems, it is often implemented with a collection of various data structures. Thus, identifying the quantity to keep track of during the simulation is very important as well as finding the right data structure for storing the data. Once the data model is defined, the next step is to bring the life to it.
1.1.2 Computing Motion
To make the waves move, we should define âtimeâ. See the following code:
1 #include <cstdio>
2
3 int main() {
4 double x = 0.0;
5 double y = 1.0;
6 double speedX = 1.0;
7 double speedY = -0.5;
8
9 const int fps = 100;
10 const double timeInterval = 1.0 / fps;
11
12 for (int i = 0; i < 1000; ++i) {(*@\label{code:basics-hello-simplewave1}@*)
13 // Update waves
14 }
15 return 0;
16 }
2
3 int main() {
4 double x = 0.0;
5 double y = 1.0;
6 double speedX = 1.0;
7 double speedY = -0.5;
8
9 const int fps = 100;
10 const double timeInterval = 1.0 / fps;
11
12 for (int i = 0; i < 1000; ++i) {(*@\label{code:basics-hello-simplewave1}@*)
13 // Update waves
14 }
15 return 0;
16 }
The code just got doubled in length, but still quite straightforward. First of all, the new variable
fps
stands for âframes-per-second (FPS)â, and it defines how many frames we want to draw for each second. If we invert this FPS value, which is seconds-per-frame, we get time interval between the two frames. Ri...