
eBook - ePub
Python for Beginners
Learn It as Easy as Pie
Yatin Bayya
This is a test
Share book
- English
- ePUB (mobile friendly)
- Available on iOS & Android
eBook - ePub
Python for Beginners
Learn It as Easy as Pie
Yatin Bayya
Book details
Book preview
Table of contents
Citations
About This Book
Whether you are an absolute beginner or an experienced programmer, learn Python programming in a simple, concise, and straightforward manner. Learn to build four smashing projects: a calculator, a drawing app, a login system, and a notes app. This book will walk you through the first steps of becoming a programmer as easy as pie.
Frequently asked questions
How do I cancel my subscription?
Can/how do I download books?
At the moment all of our mobile-responsive ePub books are available to download via the app. Most of our PDFs are also available to download and we're working on making the final remaining ones downloadable now. Learn more here.
What is the difference between the pricing plans?
Both plans give you full access to the library and all of Perlegoâs features. The only differences are the price and subscription period: With the annual plan youâll save around 30% compared to 12 months on the monthly plan.
What is Perlego?
We are an online textbook subscription service, where you can get access to an entire online library for less than the price of a single book per month. With over 1 million books across 1000+ topics, weâve got you covered! Learn more here.
Do you support text-to-speech?
Look out for the read-aloud symbol on your next book to see if you can listen to it. The read-aloud tool reads text aloud for you, highlighting the text as it is being read. You can pause it, speed it up and slow it down. Learn more here.
Is Python for Beginners an online PDF/ePUB?
Yes, you can access Python for Beginners by Yatin Bayya in PDF and/or ePUB format, as well as other popular books in Informatica & Informatica generale. We have over one million books available in our catalogue for you to explore.
Information
CHAPTER 1:
Introduction
The digital age is revolutionizing the world in terms of technology and software. Programmers provide the most useful software, enhance the world, and communicate with computers by thinking creatively.
Python is the language of choice for many of these next-generation problem-solvers. It is a powerful language whose explicit syntax allows new programmers to focus on developing software. As of 2020, Python is one of the most popular programming languages and ranks consistently at the top.
This book teaches the essential concepts and syntax of Python 3 and provides guidance for implementation with tutorials on building projects.
Getting Started
Without further ado, here is how to install Python 3 and get started creating applications.
To begin the installation, go to the âDownloadsâ page on the Python website (https://www.python.org/downloads). Select the latest stable download version (versions 3.x) for your operating system. Open the file after the download completes.
On Windows:
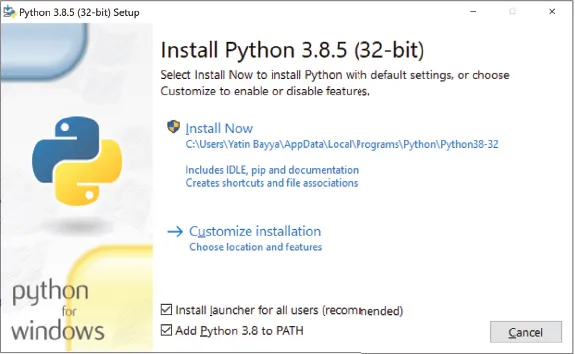
In this window, check âAdd Python 3.8 to PATHâ and click âInstall Now.â
On macOS:
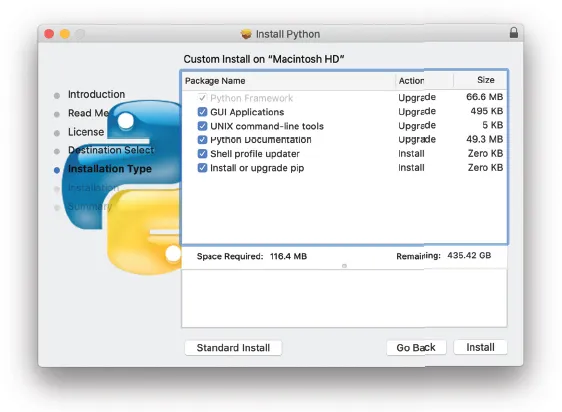
In this window, go through all the steps until âInstallation Typeâ and then click on the button âCustomizeâ and make sure that everything is selected as shown in the preceding window. After you have confirmed, everything is selected, finish the installation.
Once completed, search for the Integrated Development and Learning Environment (IDLE) application which is installed with Python earlier:
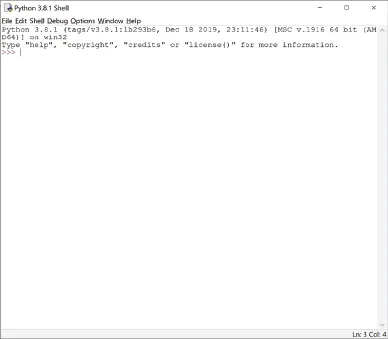
This is known as an interpreter, which evaluates and converts the code typed in there on the fly. Python is a high-level programming language that is easier for developers to read. In contrast, the computer understands the low-level machine code that is more difficult to read. The solution for the computer to understand Python is to convert this high-level code into machine code. There are several implementations (ways of converting code) to achieve this:
The first option is to use a compiler that translates the code into machine code and then runs it. The machine code created by the compiler is executable, which can be run on other computers but is not fully cross-platform compatible. The executable cannot run on any operating system unless the source code is shared, and each operating system has its own version.
Another way is to use an interpreter that translates the code on the fly and then runs it, rather than saving a separate file. The downside is that when the application needs to run on someone elseâs machine, the source code is exposed. Additionally, the client will need an interpreter to run the code. This approach is often slower than compilers because it requires to recompile every single time.
Both options have advantages and disadvantages, but the intermediate approach or bytecode combines their benefits. A virtual machine converts the source code to the lowest level possible while remaining cross-platform compatible. When running it, the compiler translates it into machine code. This code is not executed by the CPU but rather by the Python Virtual Machine (PVM). Python takes this approach, but its process is still, primarily âinterpreted,â and it also has some compilations under the intermediate approach or bytecode.
In the IDLE menu bar, click on the âFileâ button and then on the âNew Fileâ option:
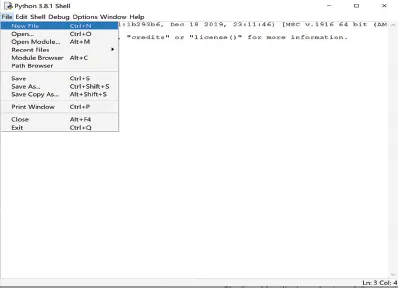
This will open a blank window for Python code. The first program will be a simple program that displays âHello Worldâ when executed:

Click on the âRunâ option and then âRun Now,â and save the file to execute the code. Now it should show the printed result of âHello World,â where commands execute and receive a result. âPrintâ is a built-in function. A function is a block of code that can be executed by using its name. Pythonâs source code defines a function to display a message and calls it to print. Essentially, the âprintâ function code outputs and displays a string (text).
The âprintâ functionâs syntax is as follows: print, parentheses, opening single/double quote, the message, the matching closing quote, closing parentheses. The message in the function must be enclosed in quotes because that differentiates code from text. Adding comments to code helps developers from a readability perspective, but the interpreter ignores them:

The octothorpe (#) denotes a comment, and all the text on the right-hand side is commented outâor not executedâand acts like plain text, doing nothing.
The code is syntax highlighted. Meaning it color codes keywords and certain distinct parts of the code, in IDLE and other text editors or integrated development environments (IDEs). Although it is possible to type Python code in any program that edits text, text editors and IDEs provide niche features. A text editor is simply a program that allows the user to type text with some syntax highlighting ability, while an IDE is similar but must also offer feature-rich programming tools for the developer.
Any text editor or IDE can be used for the purposes of this book, but, PyCharm is recommended.
Installing and Using PyCharm
PyCharm is an application that executes Python programs. Download the âCommunityâ version (https://www.jetbrains.com/pycharm/download) and follow through the installation process. Once it is installed, go through these steps:
- Create a Project.
- Give it a name.
- Be sure to uncheck the âCreate a main.py welcome script.â
- In the project:
- Check whether the interpreter is selected there:
- On Windows:
Click âFileâ on the menu and go to âSettings.â
- On macOS:
Click on âPyCharmâ on the menu and go to âPreferences.â
- Search for the âPython Interpreterâ and open it.
- Select the Python version installed:
- On Windows:
- Create a new Python file and give it a name.
- Open that file.
- In the file, call or use the print function to display âHello World.â
- Right-click and run the application.
- âHello Worldâ should appear in the bottom window.
- Check whether the interpreter is selected there:
It is recommended to create a new Project for every program you write. Each project should have related files that all come together to create a program.
Using the Interpreter
When IDLE is opened without choosing a file, it will open an interpreter, which can also be accessed by typing in âpythonâ to the Terminal (macOS/Linux) or Command Prompt (Windows) application, which should be already on your system.
The Python code, entered in the interpreter, will be evaluated or executed on the fly:
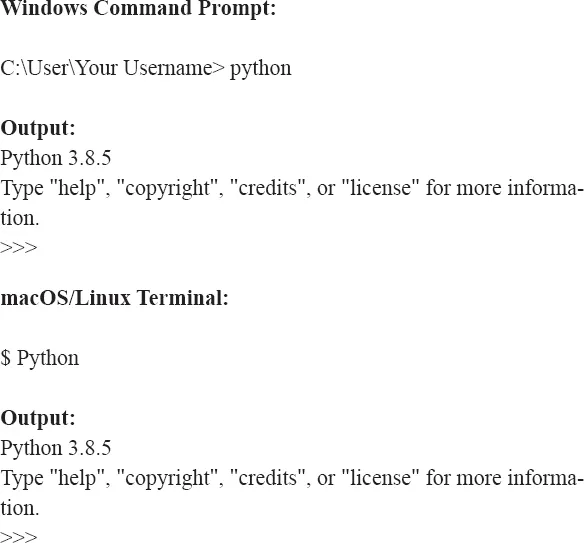
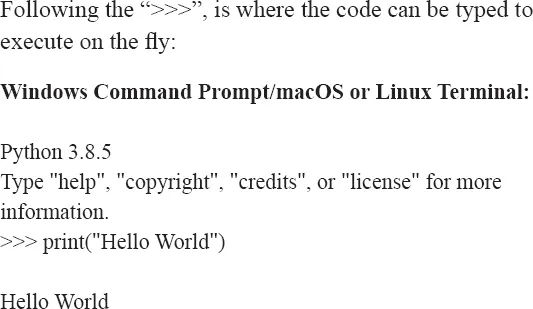
As seen in the last page, the code is executed on the fly and the output is given immediately.
CHAPTER 2:
Variables and Data
A variable is a name with an ...